Sequencer Control
The purpose of a sequencer is to allow you to programmatically control the acquisition parameters of an image sequence. You can define not only how the images are captured (i.e. the camera feature settings) but also when the camera transitions from one acquistion setting to another. This is akin to a state machine diagram where the states correspond to the sequencer set feature settings, and the transition among states corresponds to a particular event that triggers the state machine to move from one state to another.
The features to configure and deploy the sequencer are grouped under the Sequencer Control category.
Sequenced Feature Settings
The feature settings used in a sequence set are a combination of the features specifically configured for the set and what was active when the set was saved. Because many of the camera features are dependently linked to each other, settings may be saved to a set that are outside of the sequencer configuration.
Sequencer features can be classifed as follows:
Direct - direct features are those you define as being part of the set with specified values which may vary from one set to another and are independently recorded for each set. Examples include: exposure time, gain, resolution, and offset.
Implied - implied features are those that are dependent on the direct features. Their settings are recorded for each set. While you do not define their values as part of the set, they are included so that their values are valid. They can be modified during the configuration of a set, but are locked during sequencer operation.
Fixed - fixed features are those that are the preconditions to implied or direct features. These settings are recorded to guarantee the sequenced features are valid. Fixed features have a single value for the entire sequence. This is locked during sequencer configuration and operation.
Forced - forced features are those whose settings are predefined. These are automatic features that if enabled would control other features and invalidate the settings. They are forced into the Off (manual) mode during sequencer operation. During sequencer configuration, they are modifiable, to allow you to achieve the desired conditions which can then be recorded for later operation.
Non-sequenced - non-sequenced are features that are not controlled or affected by the sequencer.
The following diagram provides an example of a web of features and how they are classified based on the fact that a single feature B has been directly sequenced:
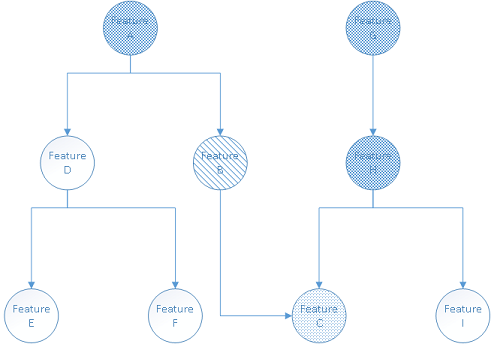
Here is an explanation of how the types of various features are resolved:
- Direct - Feature B is the only feature you have requested to be sequenced, making it the sole directly sequenced feature.
- Implied - The operational range of feature C is directly affected by feature B, hence it must be sequenced but it is implied only.
- Fixed - Feature A controls the operational range of feature B, so it must be fixed to guarantee that the recorded feature B value is legal. Feature C depends on feature H, so it must be fixed. Feature H in turn depends on feature G, so it too must be fixed.
- Non-sequenced - Features D, E, and I are not included in the sequence.
Sequencer Configuration
The configuration of a sequencer can be broken down into a number of distinct steps :
1. Enable the direct features.
Use the SequencerFeatureSelector to select which features to directly sequence and set SequencerFeatureEnable to enabled.
Enabling the direct features must be done prior to entering configuration mode as it determines those features that are defined, implied, fixed, and forced. The enabled features are active for all sequencer sets.
2. Enter configuration mode.
Set SequencerConfigurationMode to On.
When you enter configuration mode, the camera determines those features which are implied and fixed and locks the fixed features from being modified. If any fixed features need to be adjusted, exit configuration mode by setting SequencerConfigurationMode to Off. Make the necessary modifications and enter configuration mode again.
3. Configure the features for each sequencer set.
Use SequencerSetSelector to choose the set to configure. Adjust the features as needed to achieve the desired image. Use the SequencerSetSave command to save the settings to the selected sequencer set.
Once a set is saved, the camera checks that all the settings are valid. SequencerSetValid displays the results of this check. If the sequencer set is not valid, adjust the settings and save the set again.
Note: other previously saved and valid sets may become invalid when a set is saved with a new configuration.
Up to 8 sets can be configured and saved.
Once a sequencer set has been configured and is valid, it can be loaded to the active camera at anytime, just like a User Set. The difference between a User Set and a Sequencer Set is the range of features affected. You can load a sequencer set via SequencerSetLoad at anytime, not just in configuration mode.
Loading is particularly useful when trying to configure multiple sets which may share a similar setup with only minor differences. In that case, you can load an already configured set, select a different set, modify features that are different, and save.
4. Configure the transition between sets.
You must define paths for the sequencer to transition from one set to another. Use SequencerSetSelector to select the set. Then use SequencerPathSelector to select the path. The current implementation only support a single path for each set.
This path defines the event that causes the transition and the next set to which that it transitions. Use SequencerTriggerSource to specify the internal signal or physical input line to use as the trigger source. Use SequencerTriggerActivation to specify the activation mode of the selected trigger source.
Use SequencerSetNext to specify which set follows the transition.
Note: The order of steps 3 and 4 is not important, as long as the correct set has been chosen via the SequencerSetSelector.
5. Configure the starting set.
Use SequencerSetStart to define which set begins the sequencer operation when it is executed.
6. Exit configuration mode.
Set SequencerConfigurationMode to Off.
Once you have exited configuration mode, the camera checks that the sequencer configuration is valid. SequencerConfigurationValid displays the results of this check. If the configuration is not valid, enter configuration mode to adjust the settings.
Sequencer Execution
Note: Before you can execute a sequencer session, your sequencer configuration must be valid and your camera must not be streaming.
Set SequencerMode to On. This locks all sequenced features (direct, implied, fixed, and forced) and loads the starting set.
Start image acquisition. The sequencer transitions from set to set according to your path definitions. SequencerSetActive displays the current set.
During sequencer execution, all sequenced features (direct, implied, fixed, and forced) are locked as their values are driven by the sequencer. However, you can change any features that are not locked. They behave as per normal operation.
To end the sequencer session, set SequencerMode to Off. The camera is left in the same state as defined by the last sequencer set.
Sequencer Usage Example - Simple features change while acquiring images
In this example, Set 0 and Set 1 are the main sets for the camera to capture images. The directly sequenced features are Exposure Time, Gain, Width. Set 0 is defined to acquire full width images with a long exposure and no gain. Set 1 is defined to acquire half-width images with a shorter exposure and higher gain. The transition between the two sets is defined to be triggered by RisingEdge of FrameStart, meaning that the sequencer should toggle between the two sets grabbing an image based on the feature contents of the corresponding set configuration each time.
Set 0:
- ExposureTime = 4000
- Gain = 1.0
- Width = 1024
- SequencerSetTriggerSource[0] = FrameStart
- SequencerSetTriggerActivation[0] = RisingEdge
- SequencerSetTriggerSource[0] = FrameStart
- SequencerSetNext[0] = 1
Set 1:
- ExposureTime = 2000
- Gain = 2.0
- Width = 512
- SequencerSetTriggerSource[0] = FrameStart
- SequencerSetTriggerActivation[0] = RisingEdge
- SequencerSetTriggerSource[0] = FrameStart
- SequencerSetNext[0] = 0
Sequencer begins execution from Set 0.
The working diagram is shown in the following figure:
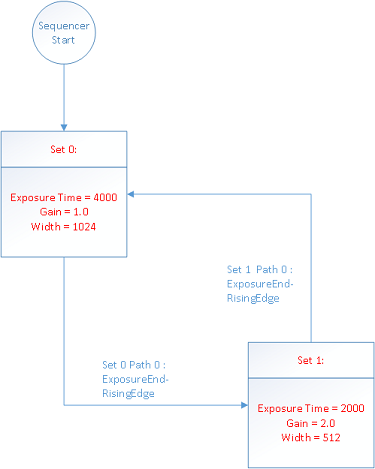
Summary Table
Name | Interface | Access | Visibility | Description |
---|---|---|---|---|
Sequencer Mode | IEnumeration | RW | Expert | Controls whether or not a sequencer is active. |
Sequencer Configuration Mode | IEnumeration | RW | Expert | Controls whether or not a sequencer is in configuration mode. |
Sequencer Configuration Valid | IEnumeration | RO | Expert | Display whether the current sequencer configuration is valid to run. |
Sequencer Configuration Reset | ICommand | RW | Expert | Resets the sequencer configuration by erasing all saved sequencer sets. |
Sequencer Feature Selector | IEnumeration | RW | Expert | Selects which sequencer features to control. |
Sequencer Feature Enable [Sequencer Feature Selector] | IBoolean | RW | Expert | Enables the selected feature and makes it active in all sequencer sets. |
Sequencer Set Start | IInteger | RW | Expert | Sets the first sequencer set to be used. |
Sequencer Set Active | IInteger | RO | Expert | Displays the currently active sequencer set. |
Sequencer Set Selector | IInteger | RW | Expert | Selects the sequencer set to which subsequent settings apply. |
Sequencer Set Valid [Sequencer Set Selector] | IEnumeration | RO | Expert | Displays whether the currently selected sequencer set's register contents are valid to use. |
Sequencer Set Save [Sequencer Set Selector] | ICommand | RW | Expert | Saves the current device configuration to the currently selected sequencer set. |
Sequencer Set Load [Sequencer Set Selector] | ICommand | RW | Expert | Loads currently selected sequencer to the current device configuration. |
Sequencer Path Selector [Sequencer Set Selector] | IInteger | RW | Expert | Selects branching path to be used for subsequent settings. |
Sequencer Trigger Source [Sequencer Set Selector] [Sequencer Path Selector] | IEnumeration | RW | Expert | Specifies the internal signal or physical input line to use as the sequencer trigger source. |
Sequencer Trigger Activation [Sequencer Set Selector] [Sequencer Path Selector] | IEnumeration | RW | Expert | Specifies the activation mode of the sequencer trigger. |
Sequencer Set Next [Sequencer Set Selector] [Sequencer Path Selector] | IInteger | RW | Expert | Specifies the next sequencer set. |
Sequencer Control Features
Sequencer Mode
Controls whether or not a sequencer is active.
Property | Value |
---|---|
Name | SequencerMode |
Interface | IEnumeration |
Access | RW |
Visibility | Expert |
Enumeration Values |
---|
Off |
On |
Sequencer Configuration Mode
Controls whether or not a sequencer is in configuration mode.
Property | Value |
---|---|
Name | SequencerConfigurationMode |
Interface | IEnumeration |
Access | RW |
Visibility | Expert |
Enumeration Values |
---|
Off |
On |
Sequencer Configuration Valid
Display whether the current sequencer configuration is valid to run.
Property | Value |
---|---|
Name | SequencerConfigurationValid |
Interface | IEnumeration |
Access | RO |
Visibility | Expert |
Enumeration Values |
---|
No |
Yes |
Sequencer Configuration Reset
Resets the sequencer configuration by erasing all saved sequencer sets.
Property | Value |
---|---|
Name | SequencerConfigurationReset |
Interface | ICommand |
Access | RW |
Visibility | Expert |
Sequencer Feature Selector
Selects which sequencer features to control.
Property | Value |
---|---|
Name | SequencerFeatureSelector |
Interface | IEnumeration |
Access | RW |
Visibility | Expert |
Enumeration Values |
---|
ExposureTime |
Gain |
OffsetX |
OffsetY |
Width |
Height |
Sequencer Feature Enable
Enables the selected feature and makes it active in all sequencer sets.
Property | Value |
---|---|
Name | SequencerFeatureEnable [Sequencer Feature Selector] |
Interface | IBoolean |
Access | RW |
Visibility | Expert |
Sequencer Set Start
Sets the first sequencer set to be used.
Property | Value |
---|---|
Name | SequencerSetStart |
Interface | IInteger |
Access | RW |
Unit | |
Visibility | Expert |
Sequencer Set Active
Displays the currently active sequencer set.
Property | Value |
---|---|
Name | SequencerSetActive |
Interface | IInteger |
Access | RO |
Unit | |
Visibility | Expert |
Sequencer Set Selector
Selects the sequencer set to which subsequent settings apply.
Property | Value |
---|---|
Name | SequencerSetSelector |
Interface | IInteger |
Access | RW |
Unit | |
Visibility | Expert |
Sequencer Set Valid
Displays whether the currently selected sequencer set's register contents are valid to use.
Property | Value |
---|---|
Name | SequencerSetValid [Sequencer Set Selector] |
Interface | IEnumeration |
Access | RO |
Visibility | Expert |
Enumeration Values |
---|
No |
Yes |
Sequencer Set Save
Saves the current device configuration to the currently selected sequencer set.
Property | Value |
---|---|
Name | SequencerSetSave [Sequencer Set Selector] |
Interface | ICommand |
Access | RW |
Visibility | Expert |
Sequencer Set Load
Loads currently selected sequencer to the current device configuration.
Property | Value |
---|---|
Name | SequencerSetLoad [Sequencer Set Selector] |
Interface | ICommand |
Access | RW |
Visibility | Expert |
Sequencer Path Selector
Selects branching path to be used for subsequent settings.
Property | Value |
---|---|
Name | SequencerPathSelector [Sequencer Set Selector] |
Interface | IInteger |
Access | RW |
Unit | |
Visibility | Expert |
Sequencer Trigger Source
Specifies the internal signal or physical input line to use as the sequencer trigger source.
Property | Value |
---|---|
Name | SequencerTriggerSource [Sequencer Set Selector] [Sequencer Path Selector] |
Interface | IEnumeration |
Access | RW |
Visibility | Expert |
Enumeration Values |
---|
Off |
FrameStart |
Sequencer Trigger Activation
Specifies the activation mode of the sequencer trigger.
Property | Value |
---|---|
Name | SequencerTriggerActivation [Sequencer Set Selector] [Sequencer Path Selector] |
Interface | IEnumeration |
Access | RW |
Visibility | Expert |
Enumeration Values |
---|
RisingEdge |
FallingEdge |
AnyEdge |
LevelHigh |
LevelLow |
Sequencer Set Next
Specifies the next sequencer set.
Property | Value |
---|---|
Name | SequencerSetNext [Sequencer Set Selector] [Sequencer Path Selector] |
Interface | IInteger |
Access | RW |
Unit | |
Visibility | Expert |